Recent Posts
Recent Comments
Link
- Today
- Yesterday
- Total
메이쁘
(JAVA) 백준 4485번 : 녹색 옷 입은 애가 젤다지? 본문
https://www.acmicpc.net/problem/4485
4485번: 녹색 옷 입은 애가 젤다지?
문제 젤다의 전설 게임에서 화폐의 단위는 루피(rupee)다. 그런데 간혹 '도둑루피'라 불리는 검정색 루피도 존재하는데, 이걸 획득하면 오히려 소지한 루피가 감소하게 된다! 젤다의 전설 시리즈의 주인공, 링크는 지금 도둑루피만 가득한 N x N 크기의 동굴의 제일 왼쪽 위에 있다. [0][0]번 칸이기도 하다. 왜 이런 곳에 들어왔냐고 묻는다면 밖에서 사람들이 자꾸 "젤다의 전설에 나오는 녹색 애가 젤다지?"라고 물어봤기 때문이다. 링크가 녹색 옷을 입
www.acmicpc.net
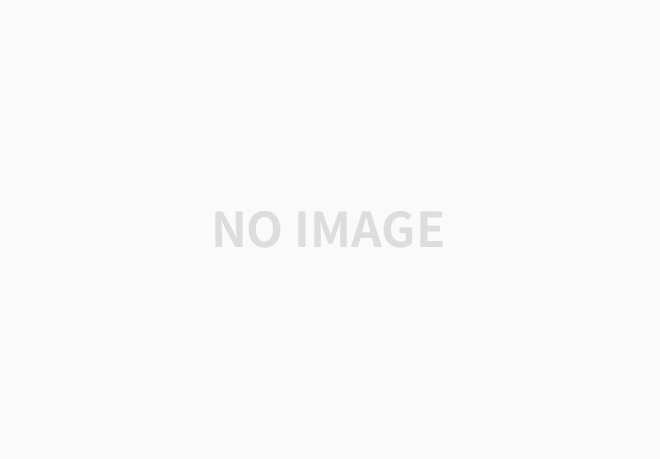
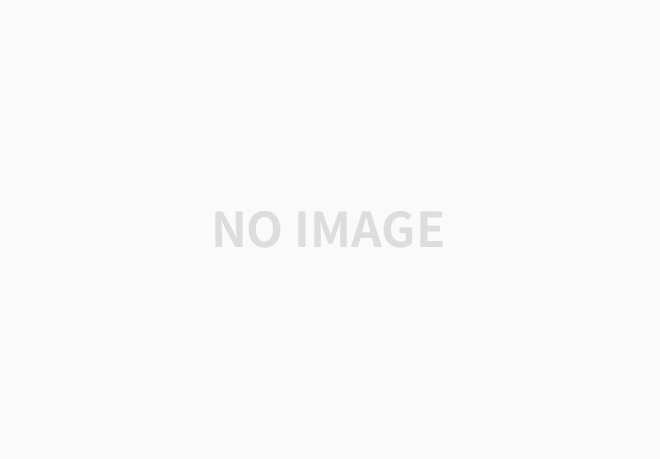
다익스트라 알고리즘 + BFS(너비우선탐색)을 섞은? 문제이다.
2차원 int 배열 map과 각 지점마다의 최단 거리를 위한 2차원 int 배열 distanceMap을 만든다.
(0, 0)에서 시작하여 상-하-좌-우로 한 번씩 이동하면서 최단 거리를 갱신한다.
** 상-하-좌-우 를 나타내기 위해 사용한 1차원 배열
// 상,하,좌,우
static int[] moveX = { 0, 0, -1, 1 };
static int[] moveY = { -1, 1, 0, 0 };
최단 거리 갱신 시에만 Queue에 넣고, Queue가 Empty되기 전까지 이를 반복한다.
*** 여기서 visit 배열을 만들 필요가 없었다..
소스코드
*** Package, import 생략
// 녹색 옷 입은 애가 젤다지? 문제
// 다익스트라 알고리즘 사용(또는 BFS도 되지 않을까?
public class p4485 {
// 상,하,좌,우
static int[] moveX = { 0, 0, -1, 1 };
static int[] moveY = { -1, 1, 0, 0 };
public static void main(String args[]) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st;
StringBuilder sb = new StringBuilder();
int count = 0;
while(true) {
st = new StringTokenizer(br.readLine());
int n = Integer.valueOf(st.nextToken());
if(n == 0) {
break;
}
count++;
int[][] map = new int[n][n]; // 0 ~ n-1 까지라고 해서
int[][] resultMap = new int[n][n];
boolean[][] visited = new boolean[n][n];
for(int i = 0; i < n; i++) {
st = new StringTokenizer(br.readLine());
Arrays.fill(resultMap[i], Integer.MAX_VALUE);
for(int j = 0; j < n; j++) {
int num = Integer.valueOf(st.nextToken());
map[i][j] = num;
}
}
int result = bfs(resultMap, map, visited, n);
sb.append("Problem ").append(count).append(": ").append(result).append("\n");
}
System.out.println(sb.toString());
}
// bfs + 다익스트라 합쳐서 사용
private static int bfs(int[][] resultMap, int[][] map, boolean[][] visited, int n) {
PriorityQueue<Point> queue = new PriorityQueue<Point>();
Point p = new Point(0, 0, map[0][0]);
resultMap[0][0] = map[0][0];
queue.add(p);
while(!queue.isEmpty()) {
Point prevPoint = queue.poll();
int x = prevPoint.x;
int y = prevPoint.y;
int weight = prevPoint.weightSum;
for(int i = 0; i < 4; i++) {
int nextX = x + moveX[i];
int nextY = y + moveY[i];
if((nextX >= 0 && nextX < n) && (nextY >= 0 && nextY < n)) {
if(weight + map[nextY][nextX] < resultMap[nextY][nextX]) {
resultMap[nextY][nextX] = weight + map[nextY][nextX];
queue.add(new Point(nextX, nextY, weight + map[nextY][nextX]));
}
}
}
}
return resultMap[n - 1][n - 1];
}
}
class Point implements Comparable<Point> {
int x;
int y;
int weightSum;
Point(int x, int y, int weightSum) {
this.x = x;
this.y = y;
this.weightSum =weightSum;
}
@Override
public int compareTo(Point o) {
if(this.weightSum < o.weightSum) {
return -1;
}
else
return 1;
}
}
이상입니다.
감사합니다!
'Algorithm > Baekjoon' 카테고리의 다른 글
(JAVA) 백준 9933번 : 민균이의 비밀번호 (0) | 2020.02.29 |
---|---|
(JAVA) 백준 2857번 : FBI (Feat. 정규표현식 정리 표) (0) | 2020.02.29 |
(JAVA) 백준 7562번 : 나이트의 이동 (0) | 2020.02.29 |
(JAVA) 백준 1120번 : 문자열 (0) | 2020.02.23 |
(JAVA) 백준 10808번 : 알파벳 개수 (0) | 2020.02.22 |